【Django】allauthを使用し、カスタムユーザーモデルを搭載させ、SendgridのAPIでメール認証をする簡易掲示板【保存版】
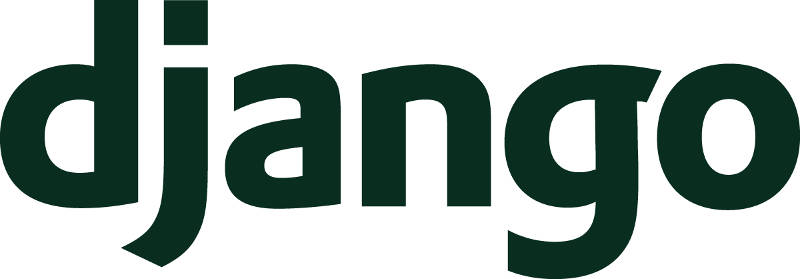
config
まず、カスタムユーザーモデルと、DjangoallauthでSendgridを使用したメール認証の設定を施す。
config/settings.py
"""
Django settings for config project.
Generated by 'django-admin startproject' using Django 3.1.2.
For more information on this file, see
https://docs.djangoproject.com/en/3.1/topics/settings/
For the full list of settings and their values, see
https://docs.djangoproject.com/en/3.1/ref/settings/
"""
from pathlib import Path
# Build paths inside the project like this: BASE_DIR / 'subdir'.
BASE_DIR = Path(__file__).resolve().parent.parent
# Quick-start development settings - unsuitable for production
# See https://docs.djangoproject.com/en/3.1/howto/deployment/checklist/
# SECURITY WARNING: keep the secret key used in production secret!
SECRET_KEY = 'al#f(6(($8m%g#l4t2-0tvv1(&hbcd+(e8dt$!-m+ospxzv0gu'
# SECURITY WARNING: don't run with debug turned on in production!
DEBUG = True
ALLOWED_HOSTS = []
SITE_ID = 1
#django-allauthログイン時とログアウト時のリダイレクトURL
LOGIN_REDIRECT_URL = '/'
ACCOUNT_LOGOUT_REDIRECT_URL = '/'
#################django-allauthでのメール認証設定ここから###################
#djangoallauthでメールでユーザー認証する際に必要になる認証バックエンド
AUTHENTICATION_BACKENDS = [
"django.contrib.auth.backends.ModelBackend",
"allauth.account.auth_backends.AuthenticationBackend",
]
#ログイン時の認証方法はemailとパスワードとする
ACCOUNT_AUTHENTICATION_METHOD = "email"
#ログイン時にユーザー名(ユーザーID)は使用しない
ACCOUNT_USERNAME_REQUIRED = "False"
#ユーザー登録時に入力したメールアドレスに、確認メールを送信する事を必須(mandatory)とする
ACCOUNT_EMAIL_VARIFICATION = "mandatory"
#ユーザー登録画面でメールアドレス入力を要求する(True)
ACCOUNT_EMAIL_REQUIRED = True
#ここにメール送信設定を入力する(Sendgridを使用する場合)
EMAIL_BACKEND = "sendgrid_backend.SendgridBackend"
"""
DEFAULT_FROM_EMAIL = "ここに送信元メールアドレスを指定"
SENDGRID_API_KEY = "ここにAPIキーを入力"
"""
SENDGRID_SANDBOX_MODE_IN_DEBUG = False
#DEBUGがTrueのとき、メールの内容は全て端末に表示させる。開発中はSendgridを使ってメール送信をするのではなく、できるだけターミナルに表示させる
if DEBUG:
EMAIL_BACKEND = "django.core.mail.backends.console.EmailBackend"
#CHECK:認証時のメールの本文等の編集は templates/allauth/account/email/ から行うことができる
#################django-allauthでのメール認証設定ここまで###################
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'users.apps.UsersConfig',
'django.contrib.sites',
'allauth',
'allauth.account',
'allauth.socialaccount',
'bbs.apps.BbsConfig',
]
AUTH_USER_MODEL = 'users.CustomUser'
ACCOUNT_FORMS = { "signup":"users.forms.SignupForm"}
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
ROOT_URLCONF = 'config.urls'
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [ BASE_DIR / "templates",
BASE_DIR / "allauth",
],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
WSGI_APPLICATION = 'config.wsgi.application'
# Database
# https://docs.djangoproject.com/en/3.1/ref/settings/#databases
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
# Password validation
# https://docs.djangoproject.com/en/3.1/ref/settings/#auth-password-validators
AUTH_PASSWORD_VALIDATORS = [
{
'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator',
},
]
# Internationalization
# https://docs.djangoproject.com/en/3.1/topics/i18n/
LANGUAGE_CODE = 'ja'
TIME_ZONE = 'Asia/Tokyo'
USE_I18N = True
USE_L10N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
# https://docs.djangoproject.com/en/3.1/howto/static-files/
STATIC_URL = '/static/'
DEFAULT_AUTO_FIELD='django.db.models.AutoField'
関連記事は下記
- 【メール認証】Django-allauthの実装方法とテンプレート編集【ID認証】
- DjangoでSendgridを実装させる方法【APIキーと2段階認証を利用する】
- 【Django】allauthとカスタムユーザーモデルを実装した簡易掲示板を作る【AbstrastBaseUser】
config/urls.py
"""config URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/3.1/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: path('', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.urls import include, path
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
"""
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include("bbs.urls"), name="bbs"),
path('accounts/', include('allauth.urls')),
]
allauthで使用するallauthに内蔵されているurls.pyを割り当てている。
カスタムユーザーモデル
user/models.py
from django.db import models
from django.contrib.auth.models import AbstractBaseUser, PermissionsMixin, UserManager
from django.contrib.auth.validators import UnicodeUsernameValidator
from django.utils import timezone
from django.utils.translation import gettext_lazy as _
from django.core.mail import send_mail
import uuid
#ここ( https://github.com/django/django/blob/master/django/contrib/auth/models.py#L321 )から流用
class CustomUser(AbstractBaseUser, PermissionsMixin):
username_validator = UnicodeUsernameValidator()
id = models.UUIDField( default=uuid.uuid4, primary_key=True, editable=False )
username = models.CharField(
_('username'),
max_length=150,
unique=True,
help_text=_('Required. 150 characters or fewer. Letters, digits and @/./+/-/_ only.'),
validators=[username_validator],
error_messages={
'unique': _("A user with that username already exists."),
},
)
first_name = models.CharField(_('first name'), max_length=150, blank=True)
last_name = models.CharField(_('last name'), max_length=150, blank=True)
#メールの入力を必須化。
email = models.EmailField(_('email address'))
is_staff = models.BooleanField(
_('staff status'),
default=False,
help_text=_('Designates whether the user can log into this admin site.'),
)
is_active = models.BooleanField(
_('active'),
default=True,
help_text=_(
'Designates whether this user should be treated as active. '
'Unselect this instead of deleting accounts.'
),
)
date_joined = models.DateTimeField(_('date joined'), default=timezone.now)
objects = UserManager()
EMAIL_FIELD = 'email'
USERNAME_FIELD = 'username'
#createsuperuserを実行し、メールアドレスを入力必須化させる。
REQUIRED_FIELDS = ['email']
class Meta:
verbose_name = _('user')
verbose_name_plural = _('users')
#abstract = True #この指定がないとカスタムユーザーモデルは反映されず、マイグレーションエラーになる。
def clean(self):
super().clean()
self.email = self.__class__.objects.normalize_email(self.email)
def get_full_name(self):
"""
Return the first_name plus the last_name, with a space in between.
"""
full_name = '%s %s' % (self.first_name, self.last_name)
return full_name.strip()
def get_short_name(self):
"""Return the short name for the user."""
return self.first_name
def email_user(self, subject, message, from_email=None, **kwargs):
"""Send an email to this user."""
send_mail(subject, message, from_email, [self.email], **kwargs)
ユーザーモデルの主キーはUUID型に仕立てた。この方がセキュリティ的に固めになる。最もユーザーIDをURLなどに含める場合はそれほどセキュリティ的に強化されるわけではないが。
user/forms.py
新規会員登録をする時にバリデーションするフィールドを指定する。
from django.contrib.auth.forms import UserCreationForm
from .models import CustomUser
class SignupForm(UserCreationForm):
class Meta(UserCreationForm.Meta):
model = CustomUser
#会員登録時、メールの入力を必須化。
fields = [ "username","email" ]
ここにemailを指定しないといけない。
user/admin.py
カスタムしたユーザーモデルを管理サイトで編集可能に仕立てる。
from django.contrib import admin
from django.contrib.auth.admin import UserAdmin
from django.utils.translation import gettext_lazy as _
from .models import CustomUser
class CustomUserAdmin(UserAdmin):
fieldsets = (
(None, {'fields': ('username', 'password')}),
(_('Personal info'), {'fields': ('first_name', 'last_name', 'email')}),
(_('Permissions'), {'fields': ('is_active', 'is_staff', 'is_superuser', 'groups', 'user_permissions')}),
(_('Important dates'), {'fields': ('last_login', 'date_joined')}),
)
#管理サイトから追加するときのフォーム
add_fieldsets = (
(None, {
'classes': ('wide',),
'fields': ('username', 'password1', 'password2'),
}),
)
list_display = ('username', 'email', 'first_name', 'last_name', 'is_staff')
search_fields = ('username', 'first_name', 'last_name', 'email')
admin.site.register(CustomUser, CustomUserAdmin)
アプリ
簡易掲示板アプリのコード
bbs/models.py
from django.db import models
from django.conf import settings
class Topic(models.Model):
comment = models.CharField(verbose_name="コメント",max_length=2000)
user = models.ForeignKey(settings.AUTH_USER_MODEL,verbose_name="投稿者",on_delete=models.CASCADE)
def __str__(self):
return self.comment
【Django】ユーザーモデルと1対多のリレーションを組む方法【カスタムユーザーモデル不使用】の方法と違って、settings.pyを読み取り、その中にある、AUTH_USER_MODEL
を1対多のリレーションを組むモデルとして指定する。
bbs/forms.py
from django import forms
from .models import Topic
class TopicForm(forms.ModelForm):
class Meta:
model = Topic
fields = [ "comment","user" ]
投稿されたコメントとユーザーをバリデーションする。
bbs/views.py
from django.shortcuts import render,redirect
from django.contrib.auth.mixins import LoginRequiredMixin
from django.views import View
from .models import Topic
from .forms import TopicForm
class IndexView(LoginRequiredMixin,View):
def get(self, request, *args, **kwargs):
context = {}
context["topics"] = Topic.objects.all()
return render(request,"bbs/index.html",context)
def post(self, request, *args, **kwargs):
copied = request.POST.copy()
copied["user"] = request.user.id
form = TopicForm(copied)
if not form.is_valid():
print("バリデーションNG")
return redirect("bbs:index")
print("バリデーションOK")
form.save()
return redirect("bbs:index")
index = IndexView.as_view()
POSTされた時、ユーザーIDを仕込む。その上でバリデーションする。
マイグレーション
必ず、userアプリからマイグレーションファイルを作る。その上でbbsアプリのマイグレーションファイルを作る。
python3 manage.py makemigrations user
python3 manage.py makemigrations bbs
python3 manage.py migrate
結論
後はSendgridに会員登録をして、APIキーを取得し、その上でsettings.pyにセットすれば、実在するメールアドレスである限り、会員登録時のメールが届く。
メールのテンプレートはallauthのテンプレートから書き換えれば良い
ソースコード
https://github.com/seiya0723/bbs_allauth_sendgrid_custom_user_model